74. Search a 2D Matrix
Write an efficient algorithm that searches for a value in an m x n matrix. This matrix has the following properties:
- Integers in each row are sorted from left to right.
- The first integer of each row is greater than the last integer of the previous row.
1
2
3
4
| Example 1:
Input: matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]], target = 3
Output: true
|
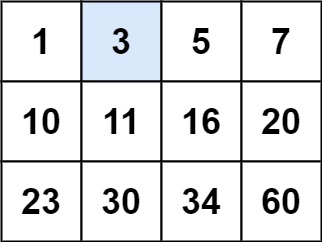
1
2
3
4
| Example 2:
Input: matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]], target = 13
Output: false
|
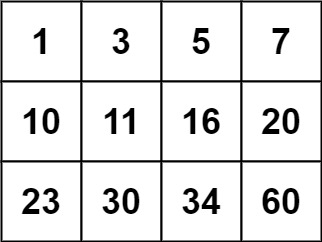
Constraints:
- m == matrix.length
- n == matrix[i].length
- 1 <= m, n <= 100
- -104 <= matrix[i][j], target <= 10^4
Solution#
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
| class Solution {
public boolean searchMatrix(int[][] matrix, int target) {
int cols = matrix.length;
int rows = matrix[0].length;
int lo = 0;
int hi = cols * rows - 1;
while (lo <= hi) {
int mid = lo + (hi - lo) / 2;
int value = matrix[mid / rows][mid % rows];
if (value == target) {
return true;
}
if (target < value) {
hi = mid - 1;
} else {
lo = mid + 1;
}
}
return false;
}
}
|