255. Verify Preorder Sequence in Binary Search Tree
Given an array of unique integers preorder, return true if it is the correct preorder traversal sequence of a binary search tree.
1
2
3
4
5
6
7
8
9
| Example 1:
Input: preorder = [5,2,1,3,6]
Output: true
Example 2:
Input: preorder = [5,2,6,1,3]
Output: false
|
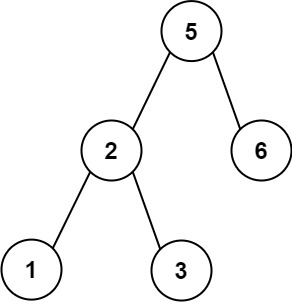
Constraints:
- 1 <= preorder.length <= 10^4
- 1 <= preorder[i] <= 10^4
- All the elements of preorder are unique.
Solution#
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
| class Solution {
public boolean verifyPreorder(int[] preorder) {
Stack<Integer> stack = new Stack<>();
int lowerBound = Integer.MIN_VALUE;
for (int val: preorder) {
while (stack.size() > 0 && val > stack.peek()) {
lowerBound = stack.pop();
}
if (val < lowerBound) {
return false;
}
stack.push(val);
}
return true;
}
}
|